In times when we lack inspiration, it might help to have a program randomly picking an aphorism for us. An aphorism is a concise expression of a general truth or principle. But you can also use a joke or anything else.
Let's see how we can implement this with desktop notifications, python, and cron.
Requirements
You will need these
- python3 with notify2 library. Install it with
pip install notify2
- a notification daemon. I use and recommend dunst if you are on Linux and want a full-featured and lightweight daemon.
- A way to run your script periodically. A popular way under Linux is to use Cron, which has various implemetations. I recommend the package cronie.
Once you have all of them installed, you will need to run dunst by typing dunst
in a terminal. Also start cronie. In Archlinux do systemctl start cronie
.
With all these points checked, we are ready to script!
Python script
Notify2 is quite simple to use. If you want try sending a notification, use
import notify2
notify2.init("Program name") # Define the name of your program which will send notifications
n = notify2.Notification("Hello world!")
n.show()
You should see appearing a notification on your desktop.
Now that we know how to notify, let's extract a random aphorism. You may populate a file called aphorisms.txt
with a handful of aphorisms.
Actions speak louder than words.
Give a man a fish and you feed him for a day; teach a man to fish and you feed him for a lifetime.
You can kill a man but you can't kill an idea.
Genius is one percent inspiration and 99 percent perspiration.
Measure twice cut once.
To extract a random aphorism from this file, we will read all lines of the file, and select one line randomly.
import random
with open("aphorisms.txt", mode='r') as f:
aphorisms = f.readlines()
choice = random.randint(1,len(aphorisms) - 1)] # Choose one line randomly.
aphorism = aphorisms[choice].strip()
Use .strip()
to remove the trailing \n
character which is means "new line" when reading the file.
Last thing we have to tell our script which D-Bus address to use. D-Bus is the program in Linux which enable programs to interact. By telling which D-Bus address to use, the script will successfully reach the notification daemon running on your graphical user session. Enough with complicated stuff, you just have to write:
import os
os.environ['DBUS_SESSION_BUS_ADDRESS'] = 'unix:path=/run/user/1000/bus'
unix:path=/run/user/1000/bus
may differ in your case. To check your settings, open a terminal and run echo $DBUS_SESSION_BUS_ADDRESS
, then copy the exact output of the command instead.
We finally obtain the following code. I used functions to make it easily understandable.
#!/usr/bin/python
# -*- coding: utf-8 -*-
import notify2
import random
import os
os.environ['DBUS_SESSION_BUS_ADDRESS'] = 'unix:path=/run/user/1000/bus'
def get_aphorisms(path):
with open(path, mode='r') as f:
return f.readlines()
notify2.init('Random aphorism')
def notify(message):
notify2.Notification(message).show()
def random_aphorism(path):
aphorisms = get_aphorisms(path)
return aphorisms[random.randint(1,len(aphorisms) - 1)].strip()
if __name__=="__main__":
path = "/path/to/your/data/aphorisms.txt"
aphorism = random_aphorism(path)
notify(aphorism)
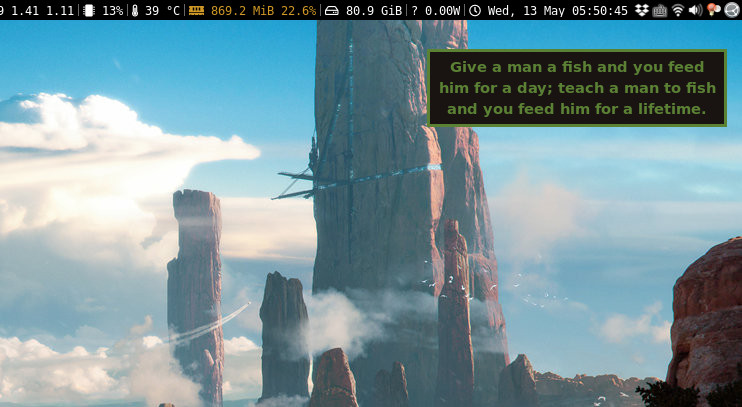
The notification displays on the desktop. The specific display is due to dunst with my configuration file.
Regular notifications
Let's now display it every three hours. We make a cron job with cronie, by running crontab -e
. It should open your text editor, defined by the environment variable $VISUAL
, and defaults to vi. In the file to edit, put
0 */3 * * * /bin/python /path/to/your/script/aphorism.py
Add at least one blank line at the end of the file or crontab will complain about "premature EOF". Be careful about spelling as Cron won't tell you whether your command failed.
Improvements
That's all for today! You can improve the code by adding the author of the aphorism, or quote, using notify2.Notification(message, author).show()
instead. The complete code is also available on my github.